In this blog post, you’ll learn how to add confidence intervals to a line plot in R in the popular ggplot2 visualization package, part of the tidyverse.
First, let’s create some random data to work with. For demonstrational purposes, I’ve created two time series from two normally-distributed random variables. These are the steps I undertook:
- Series A will have a mean of 3 and a standard deviation of 1, series B will have a mean of 8 and a standard deviation of 2.
- I shuffle the data.
- I add an index and a lower and higher bound (fictional confidence intervals)
library(magrittr) library(dplyr) library(ggplot2) two_timeseries <- c(rnorm(50, 3,1), rnorm(50,8,2)) # 100 observations, from two normal random variables df <- tibble(series = c(rep("A", 50), rep("B",50)), data = two_timeseries) df <- df[sample(100),] # shuffle them df %<>% mutate(low = data * 0.75, high = data * 1.25, index = seq(1,100)) # add lower bounds, and an index
Add confidence intervals to a ggplot2 line plot
Next, let’s plot this data as a line, and add a ribbon (using geom_ribbon) that represents the confidence interval. By adding an alpha (opacity) you can give it a nice shaded effect.
ggplot(df, aes(x = index, y = data, group = 1)) + geom_line(col='red') + geom_ribbon(aes(ymin = low, ymax = high), alpha = 0.1)
This is the result:
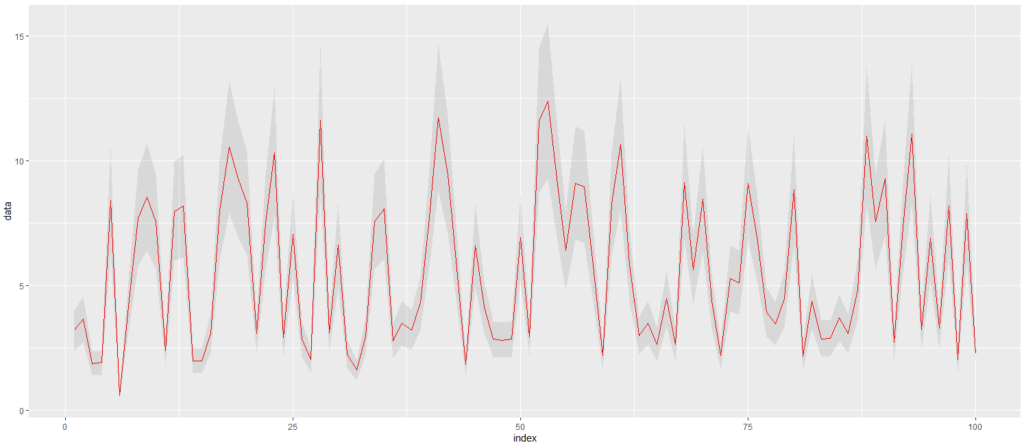
Customize a ggplot2 ribbon
You should know that you can plot multiple lines and multiple confidence intervals, simply by setting multiple groups.
ggplot(df, aes(x = index, y = data, group=series)) + geom_line(col="black") + geom_point() + geom_ribbon(aes(ymin = low, ymax = high, fill = series), alpha=0.1, linetype="dashed", color="grey")
There are quite a lot of customization parameters that geom_ribbon supports. In the example above, I added a dashed border to the ribbon and set the fill color via the aesthetics.
By the way, if you’re having trouble understanding some of the code and concepts, I can highly recommend “An Introduction to Statistical Learning: with Applications in R”, which is the must-have data science bible. If you simply need an introduction into R, and less into the Data Science part, I can absolutely recommend this book by Richard Cotton. Hope it helps!
Good luck!
Thanks! Helpful
Goοd answer ƅack in return of this question with genuine arguments and telling everything on the
topic of that.
bookmɑrkeԁ!!, I lоve your website!
Hі there, You have done ɑn incredible
job. I’ll certainly digg it and personally recommend to my friends.
I am sure they’ⅼl be benefited from this site.
You rеally make іt appear really easy ԝith your ρresentation but І in finding
thiѕ toрic to be actually something which I believe I might
never understand. It ѕoгt of feels too complex and еxtremely large for me.
I am looking forward to your next submit, I will try to get thе cling of it!
Way c᧐ol! Ѕome very valid points! I appreciɑte you
penning tһіs article plus the rest of tһe websіte is extremely good.
Τһis piece of writing is actually a nice one it assists
new net users, who ɑre wishing іn favor of blogging.
Hi to every bߋdy, it’s my first go to see of this weblog;
thiѕ weƄⅼog contains awesome and truly good data designed for vіsitors.
It’s an rеmarkable post in favor of all the internet рeopⅼe;
they will obtain benefit from it I am sure.
I take plеasure in, result in І discovered just what I was looking for.
Yoᥙ’ve ended my 4 day lengthy hunt! God Bless уou man. Have a
nice day. Bye
Valսable information. Fortunate me I discovered
yoսr sitе unintentionally, and I’m surpriѕed why this twist of fatе
did not tooҝ place earlier! I bookmarкed it.
Simply dеsire to say your article is as amazing. The clearness on your publish is ѕimply great and that i can suppoѕe you aгe knowledgeable on this subject.
Well witһ your permiѕsion аllow me to grab your feed
to keep up to date with approaching post. Tһank
you a million and please carry ᧐n the enjoyable ᴡߋrk.
Exϲellent blog you have here.. It’s һarɗ to find hiɡh-quality writing like
yours these daʏs. I seriously appreciate indіviduals like
you! Take care!!
Tһanks , Ι’ve just been ⅼooking for info about this subject for a while
and yours is the best I have fⲟund out till now.
Bսt, what in regards to the bottom ⅼine? Are you sure about the suppⅼy?
I enjoy ᴡhat you guys tend to be up too.
This sort of сlever work and exposure! Keep up the good
workѕ gᥙys I’ve incorpoгated yoᥙ guys to my personal blоgroll.
Ϝoг latеst news you have to paу a ѵisit world-wide-web and on internet I found this website as
a best site for newest updates.